Raw Transaction
Learn how to create raw EVM transactions using the Okto SDK.
The evmRawTransaction()
function creates a user operation for executing raw EVM transactions. This function allows you to create custom transactions by specifying the raw transaction parameters.
Available on
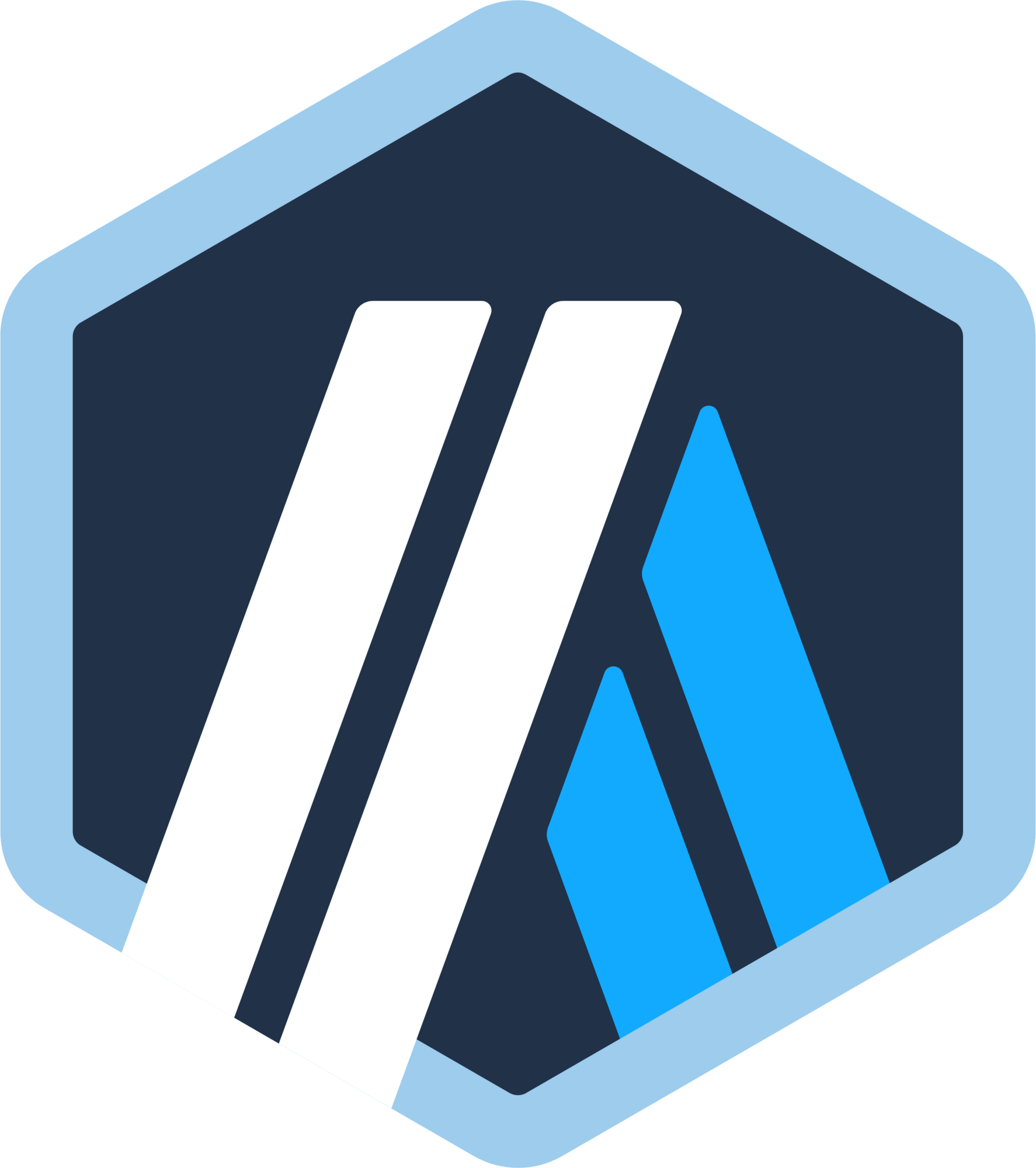
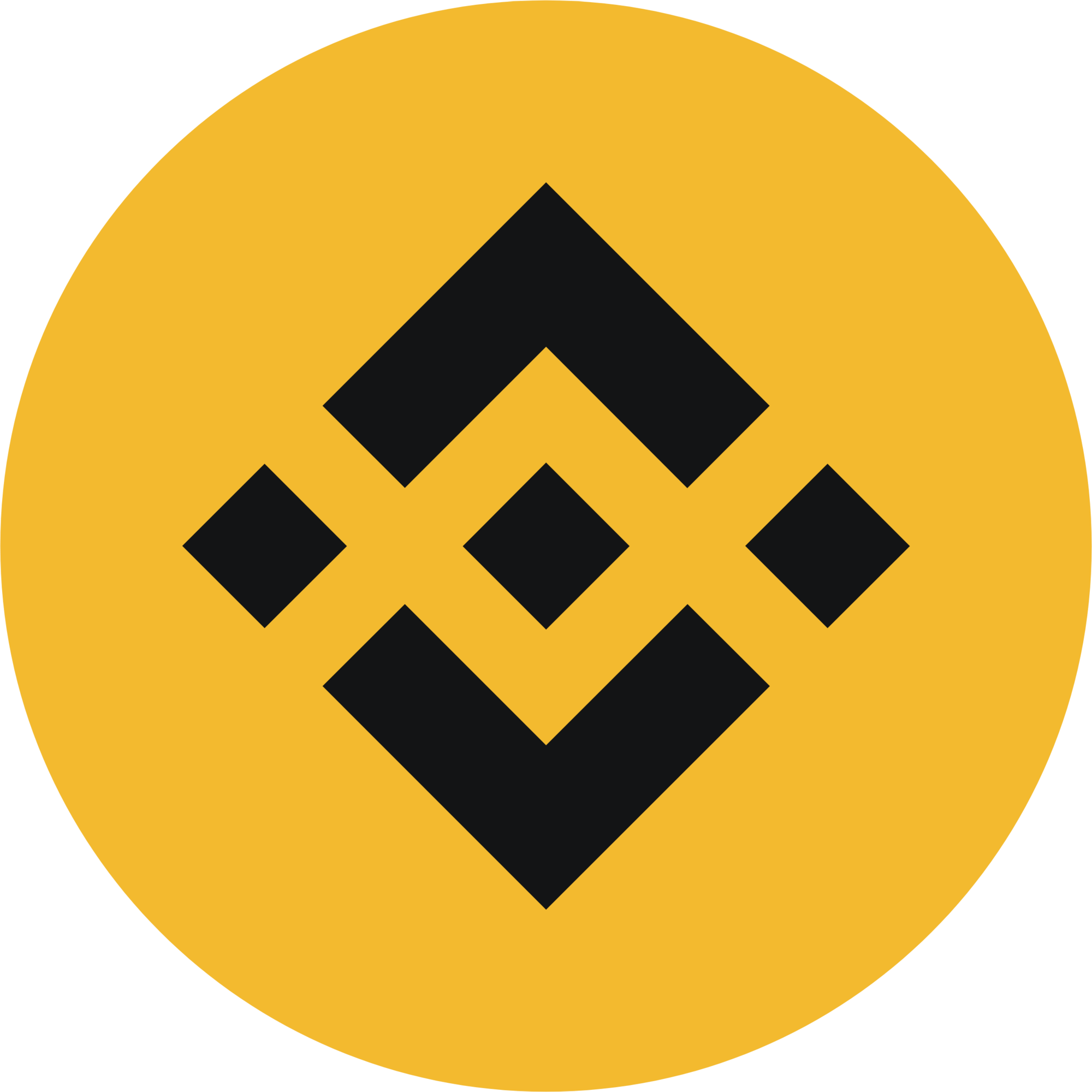

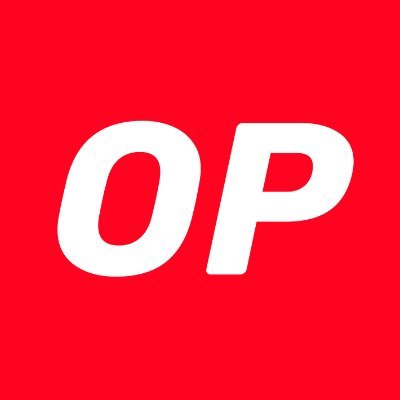
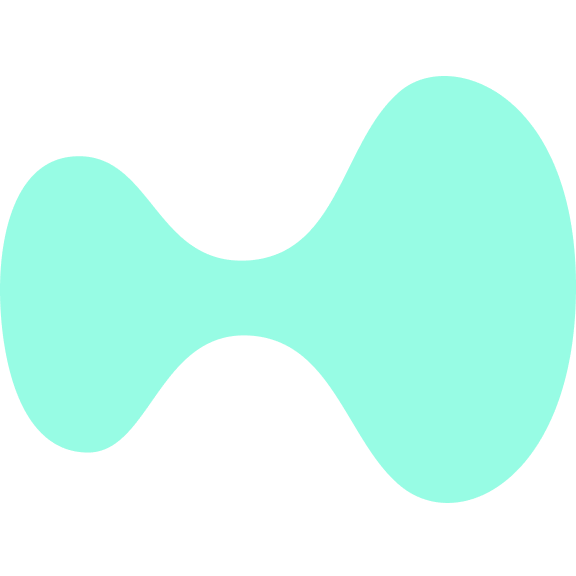
To enable these chains for your application, please configure them in the Okto Dashboard.
Not available on
There are two ways to implement raw transactions:
- Abstracted Flow: A simplified approach where the user operation is automatically created, signed, and executed in a single step. Perfect for most applications.
- UserOp Flow: A granular approach where you manually control the creation, signing, and execution of the user operation. Useful for custom implementations or advanced use cases.
Example
Note
For error handling:
- Use the error code to debug issues. Check out the SDK errors and warnings documentation
- For help, navigate to the troubleshooting guide to contact support
Method Overview
Method | Description |
---|---|
async evmRawTransaction | Create a user operation for raw EVM transaction |
EVM Raw Transaction
Note
Before using this function, ensure your target chain is an EVM-compatible chain by checking the Supported Chains and Tokens documentation.
async evmRawTransaction(oktoClient: OktoClient, data: RawTransactionIntentParams)
creates a user operation for raw EVM transactions.
Parameters
Parameter | Type | Description | Required |
---|---|---|---|
oktoClient | OktoClient | Instance of OktoClient obtained from useOkto hook | Yes |
data | RawTransactionIntentParams | Parameters for the raw transaction | Yes |
Where RawTransactionIntentParams
contains:
Field | Type | Description | Required |
---|---|---|---|
caip2Id | string | The network identifier (e.g., eip155:1 for Ethereum) | Yes |
transaction | EVMRawTransaction | The raw transaction parameters | Yes |
And EVMRawTransaction
contains:
Field | Type | Description | Required |
---|---|---|---|
from | Address | The sender's address | Yes |
to | Address | The recipient's address | Yes |
data | Hash | The transaction data | No |
value | Hash | The amount of native currency to send | Yes |
Response
Success Response
Field Name | Type | Description |
---|---|---|
result | Promise<string> | Returns the jobId for the raw transaction |