Explorer
Get Portfolio
Learn how to retrieve portfolio information using the Okto SDK.
The getPortfolio
function retrieves the user's portfolio information, including token balances and total portfolio value across all supported chains.
Available on
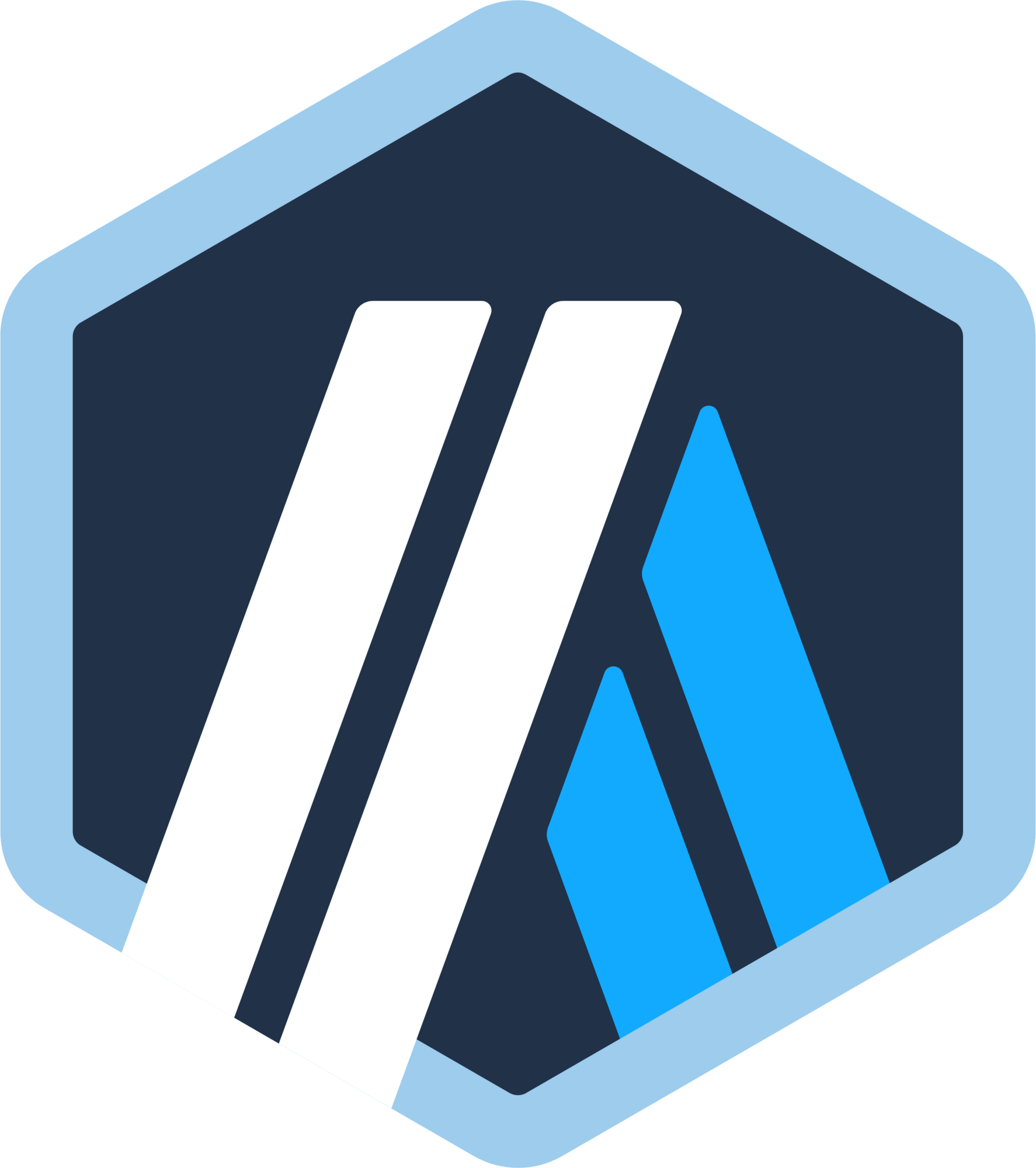
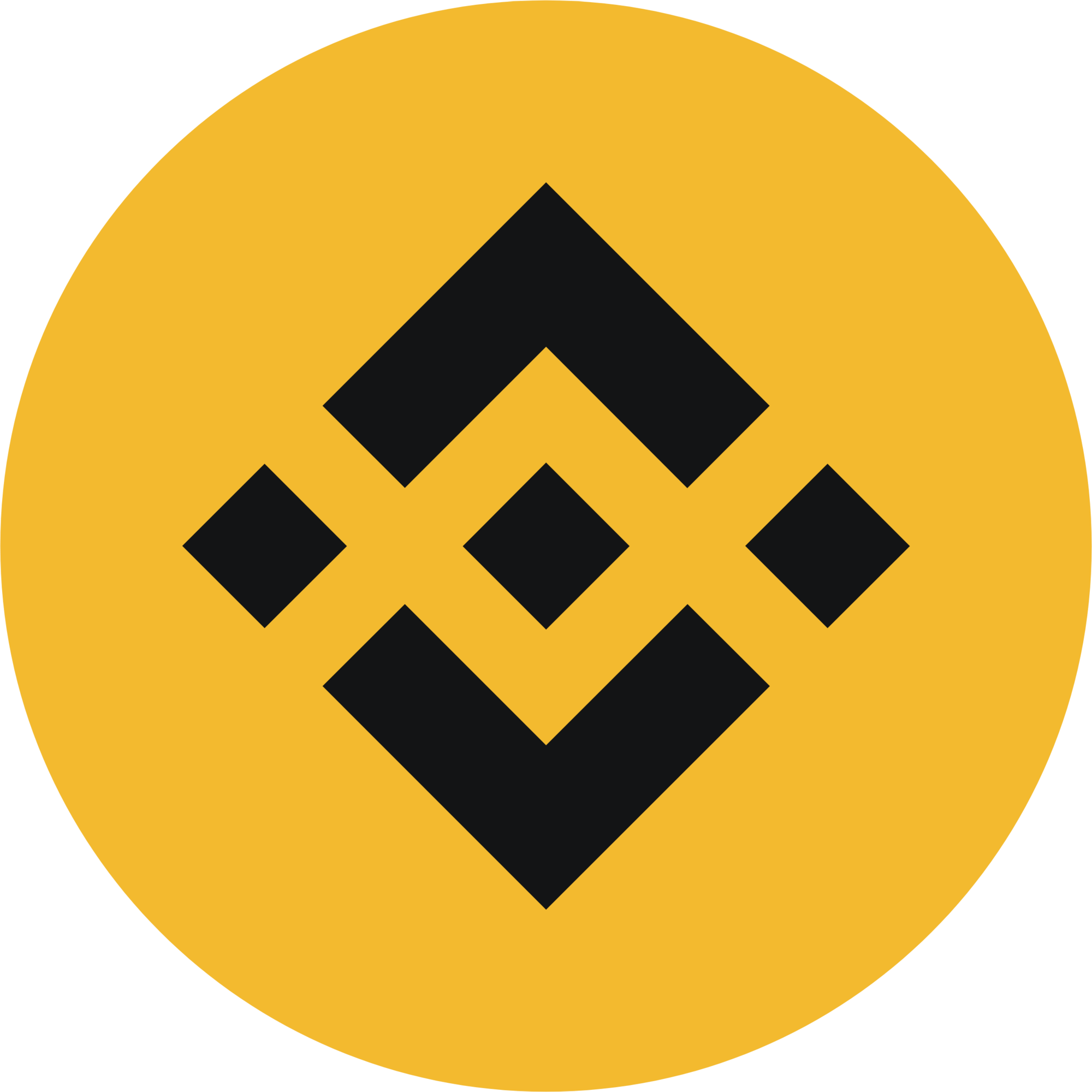

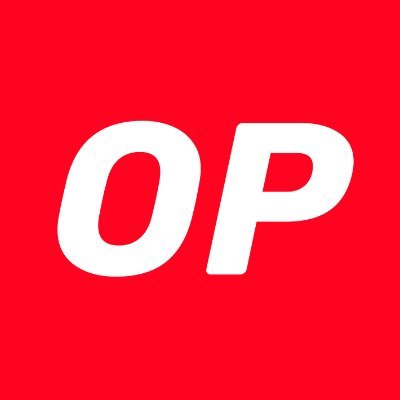
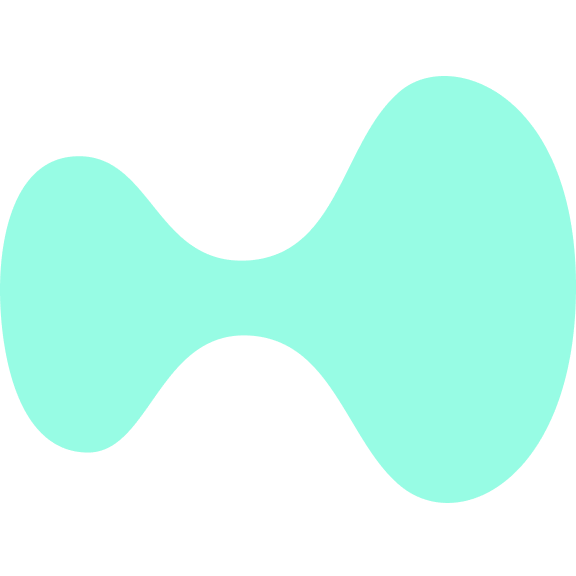
To enable these chains for your application, please configure them in the Okto Dashboard.
Not available on
Example
Note
For error handling:
- Use the error code to debug issues. Check out the SDK errors and warnings documentation
- For help, navigate to the troubleshooting guide to contact support
Method Overview
Method | Description |
---|---|
async getPortfolio | Get portfolio information for the user |
Get Portfolio
async getPortfolio(oktoClient: OktoClient)
retrieves the user's aggregated portfolio data.
Parameters
Parameter | Type | Description | Required |
---|---|---|---|
oktoClient | OktoClient | Instance of OktoClient obtained from useOkto hook | Yes |
Response
Success Response
Field Name | Type | Description |
---|---|---|
portfolio | Promise<UserPortfolioData> | User's portfolio information |