Intents
NFT Transfer
Learn how to create NFT transfer operations using the Okto TypeScript SDK.
The nftTransfer()
function creates a user operation for transferring NFTs. This function initiates the process of transferring an NFT by encoding the necessary parameters into a User Operation, which can then be signed and executed using the OktoClient.
Available on
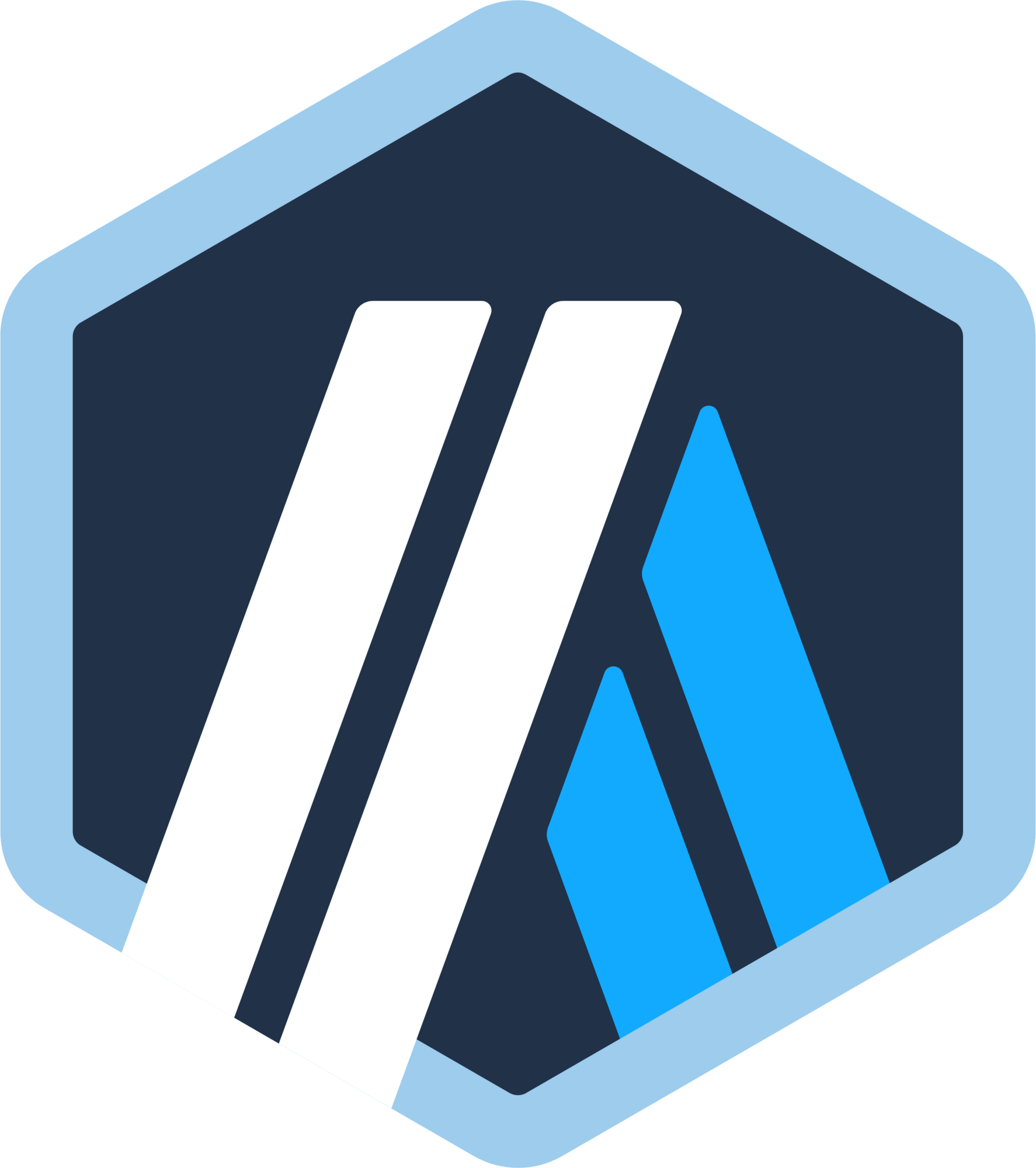
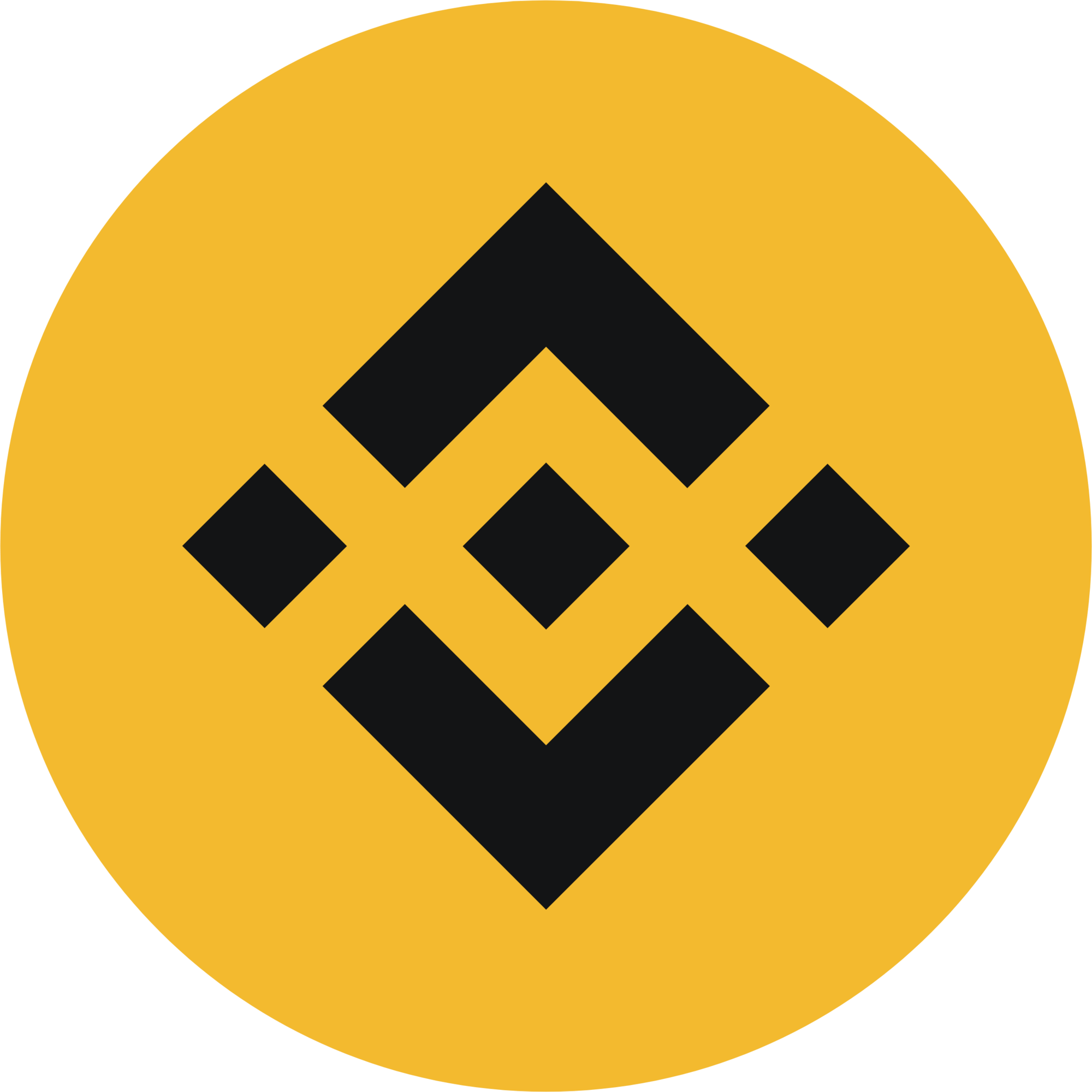

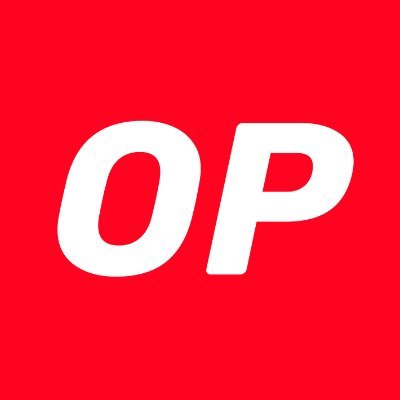
To enable these chains for your application, please configure them in the Okto Dashboard.
Not available on
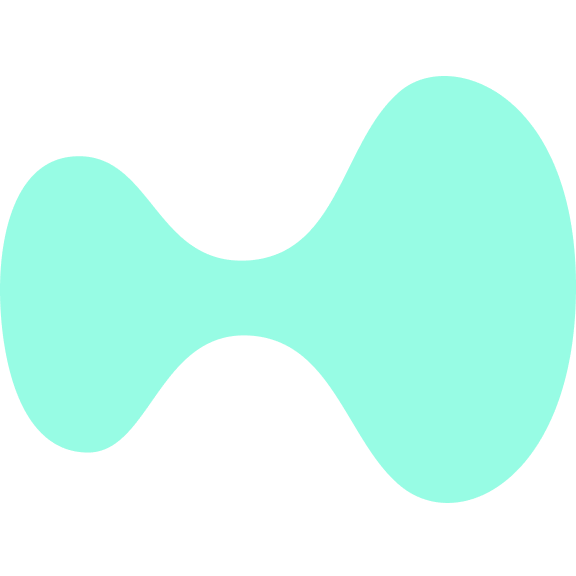
There are two ways to implement NFT transfers:
- Abstracted Flow: A simplified approach where the user operation is automatically created, signed, and executed in a single step. Perfect for most applications.
- UserOp Flow: A granular approach where you manually control the creation, signing, and execution of the user operation. Useful for custom implementations or advanced use cases.
Example
Note
For error handling:
- Use the error code to debug issues. Check out the SDK errors and warnings documentation
- For help, navigate to the troubleshooting guide to contact support
Method Overview
Method | Description |
---|---|
async nftTransfer | Create a user operation for NFT transfer |
NFT Transfer
async nftTransfer(oktoClient: OktoClient, data: NFTTransferIntentParams)
creates a user operation for transferring NFTs.
Parameters
Parameter | Type | Description | Required |
---|---|---|---|
oktoClient | OktoClient | Instance of OktoClient | Yes |
data | NFTTransferIntentParams | Parameters for the NFT transfer | Yes |
Where NFTTransferIntentParams
contains:
Field | Type | Description | Required |
---|---|---|---|
caip2Id | string | The network identifier, formatted as a CAIP network ID | Yes |
collectionAddress | Address | The address of the NFT collection | Yes |
nftId | string | The unique identifier of the NFT | Yes |
recipientWalletAddress | Address | The wallet address of the recipient | Yes |
amount | number | bigint | The amount of NFTs to transfer, typically "1" | Yes |
nftType | 'ERC721' | 'ERC1155' | The type of NFT standard | Yes |
Response
Success Response
Field Name | Type | Description |
---|---|---|
result | Promise<string> | Returns the jobId for the NFT transfer |